I needed something to feel good about, so I decided to set up Jasmine BDD spec execution in the terminal on OS X.
I downloaded the standalone zip file from Jasmine's web site and made sure that the sample specs are executing fine with the SpecRunner.html file in the browser.
I wanted to execute the exact same specs but instead of running it in the browser, I wanted to do it in terminal.
Michael Hines' blog post was a pretty good starting point. He used JazzMoney, so I tried it myself.
I easily found JazzMoney's installation instructions on their github page.
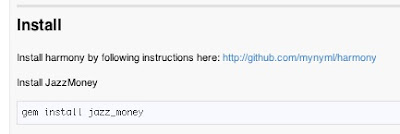
It has prerequisites: I had to install harmony first.
I picked ruby-1.9.2-head from RVM, created a new gemset called "jasmine" and I got started.
Harmony has dependencies as well, I had to get stackdeck and johnson before I installed harmony.
$ gem install stackdeck $ gem install johnson -v "2.0.0.pre3"This is where it turned ugly. Stackdeck got installed fine, but johnson had some issues.
Building native extensions. This could take a while...
ERROR: Error installing johnson:
ERROR: Failed to build gem native extension.
ERROR: Error installing johnson:
ERROR: Failed to build gem native extension.
After Googling the error I found out that johnson is not playing nice with Ruby beyond 1.8.7. I went back to RVM and started out by installing a new version of Ruby.
Here is what I did:
$ rvm install 1.8.7-p249 $ rvm 1.8.7-p249 $ rvm gemset create jasmine $ rvm 1.8.7-p249@jasmine // switched to jasmine gemset $ gem install stackdeck $ gem install johnson -v "2.0.0.pre3" $ gem install harmony // I tested harmony with a quick test in IRB, worked fine $ gem install jazz_moneyI did not have any problems with installing the gems under 1.8.7.
I had to create a Ruby script that sets up the test suite and this is the file I ran in the terminal. My run_specs.rb file was placed into the root folder right next to SpecRunner.html:
require 'rubygems' require 'jazz_money' javascript_files = [ 'spec/SpecHelper.js', 'spec/PlayerSpec.js' ] jasmine_spec_files = [ 'src/Player.js', 'src/Song.js' ] JazzMoney::Runner.new(javascript_files, jasmine_spec_files).callI ran the file with the following parameters:
$ ruby run_specs.rb -f n -cSuccess! This is the output I received in the terminal:
I did one more thing: I created a shell script, this way I did not have to remember all the command line arguments.
I saved this in the specrunner.sh file:
ruby run_specs.rb -f n -cI can now invoke my specs by running "sh specrunner.sh" in the terminal.